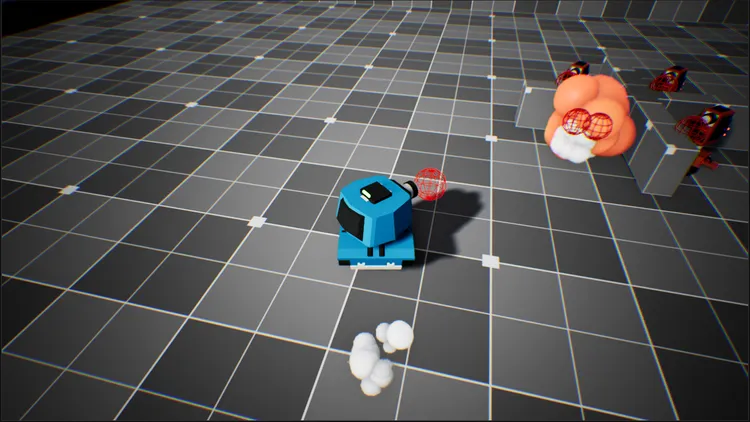
UE5Learn Gameplay (2) Toon Tanks
Unreal Engine
Overview Video
What I Learnt?
AGameModeBase
and UWorld
class
In my current thinking, they are both unique and similar to a singleton instance.
AGameModeBase
The AGameModeBase is a class employed to define game rules and settings. It typically handles the following aspects:
-
Game Rules: By inheriting AGameModeBase, I can create customized game modes to delineate game rules and logic, such as determining the player’s life value and winning conditions.
-
Player Spawn Points: It also enables the definition of player spawn points in the game world.
-
Game State: It keeps track of the game state, detailing if the game has commenced or concluded.
-
Player Controller: The class allows for the specification of a player controller class tasked with handling player inputs.
In this project, I configured a game mode using C++ that will be utilized throughout the game. Within this framework, I outlined the game’s delay onset time and instances of Pawns, including controls for both the player and enemies.
// Control the end contidition of the game
void AToonTankGameMode::ActorDied(AActor* DeadActor)
{
if (DeadActor == TankRef)
{
TankRef->HandleDestruction();
if (TankPlayerControllerRef)
{
TankPlayerControllerRef->SetPlayerEnabledState(false);
}
GameOver(false);
}
else if (ATower* DestroyedTower = Cast<ATower>(DeadActor))
{
DestroyedTower->HandleDestruction();
TargetTowers--;
if (TargetTowers == 0)
{
GameOver(true);
}
}
}
Subsequently, by registering and executing events adorned with
the UFUNCTION(BlueprintImplementableEvent)
modifier in Blueprint,
I achieved control over the UI Widget.
Consequently, it abides by the principles of the singleton pattern, existing uniquely within a specific game instance.
UWorld
The UWorld
object acts as a container for all game objects such as characters, artifacts, etc.
In UE5, one can directly obtain the world object through:
GetWorld()
It primarily encompasses the following elements and functionalities:
- Object Container: It harbors and manages all game entities including characters, NPCs (Non-Player Characters), and static and dynamic environment objects.
- Environment Settings: It contains settings and attributes such as lighting and weather conditions.
- Physical Simulation: It governs physical simulations managing all physical interactions and collision detections.
- Level Management: The UWorld supervises game levels, enabling the loading, unloading, and transition between various game levels. In this project, I registered the OnDamaged callback in the HPComponent through:
GetOwner()->OnTakeAnyDamage.AddDynamic(this, &UHPComponent::OnDamageTaken);
During the instance collision of the Project, a signal is “emitted”:
auto DamageTypeClass = UDamageType::StaticClass();
if (OtherActor != nullptr && OtherActor != this && OtherActor != MyOwner)
{
UGameplayStatics::ApplyDamage(OtherActor, Damage, MyOwnerInstigator, this, DamageTypeClass);
//...
}
//...
Here, the Damage seemingly embodies an implementation of the observer and mediator patterns, with UWorld serving a mediator role encapsulating entities in a container.